React js Learning
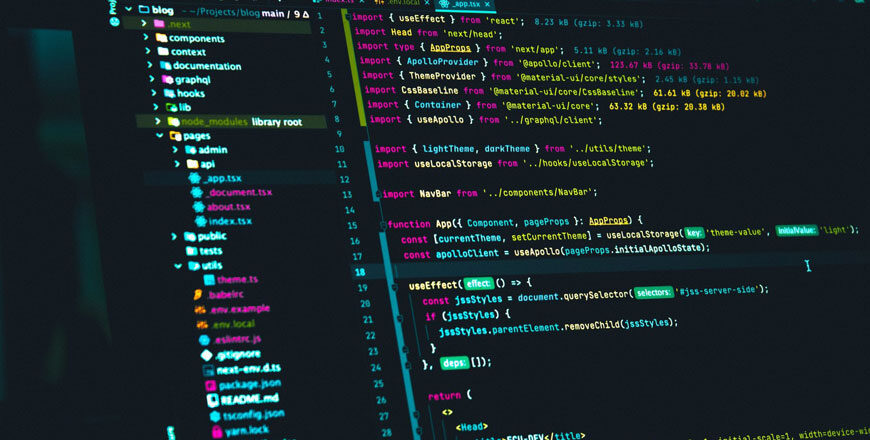
Starting Course
1
Quiz: Mobile / Native Apps
2
Engine Target Audience
After Intro
The Tensor Core GPU Architecture designed to Bring AI to Every Industry. Equipped with 640 Tensor Cores, Volta delivers over 100 teraflops per second (TFLOPS) of deep learning performance, over a 5X increase compared to prior generation NVIDIA Pascal architecture.
2
Deep Learning
Productivity Hacks to Get More Done in 2018
— 28 February 2017
- Facebook News Feed Eradicator (free chrome extension) Stay focused by removing your Facebook newsfeed and replacing it with an inspirational quote. Disable the tool anytime you want to see what friends are up to!
- Hide My Inbox (free chrome extension for Gmail) Stay focused by hiding your inbox. Click "show your inbox" at a scheduled time and batch processs everything one go.
- Habitica (free mobile + web app) Gamify your to do list. Treat your life like a game and earn gold goins for getting stuff done!